Getting Started with iOS and Xcode
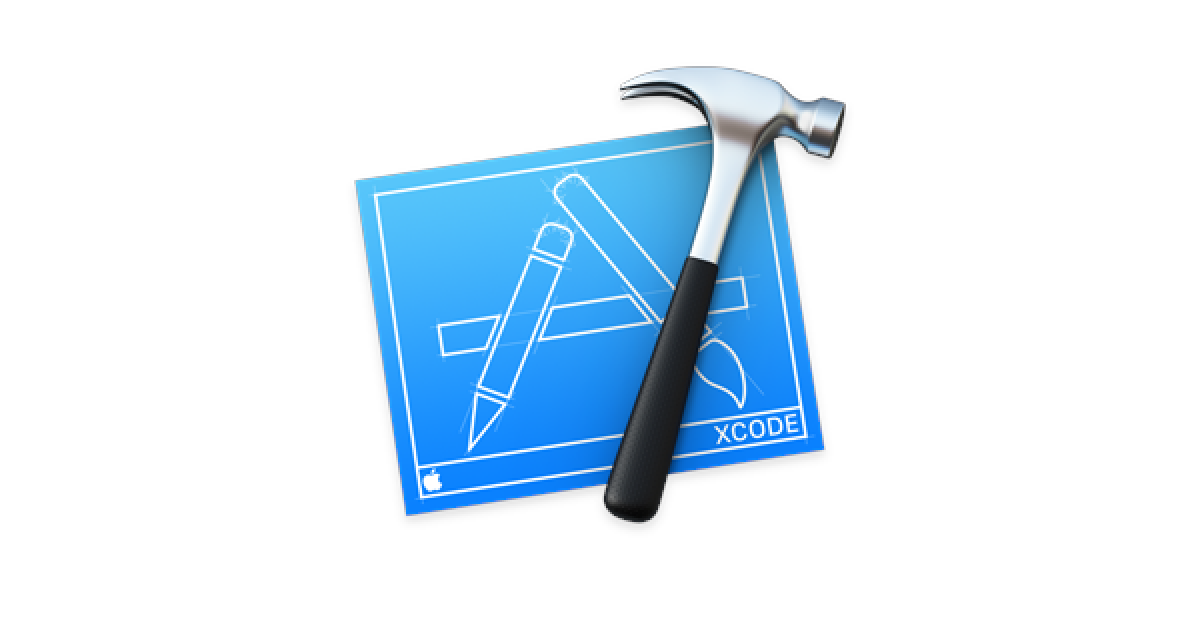
In this article, we’re going to cover not just how to get started with an iOS app, but also some good practices I’ve recently picked up to set up our project before we start writing any code. It’s fun to jump right into a new idea and start writing code, but setting up our project from the beginning can help boost our speed and productivity down the road.
There are plenty of articles out there that go over the simple steps for creating an iOS app. When I first started playing around with Xcode and making iOS apps, the first thing I’d do after creating a new project was go straight to the Main.storyboard
file and start adding view controllers and objects to those view controllers. I thought, “I’ll design my app concept first and then write the code to connect everything and make it work after I’m happy with my design.” I’d end up not getting very far and even though overtime my development startup process changed, I would never reach the final product. My architecture never made sense and my code and view controllers often violated the Don’t Repeat Yourself (DRY) principle and probably all of the S.O.L.I.D. principles (more on those in a later article). Not to mention my code was never tested and my commits were illogical. Fast-forward some time, I spent a year as a professional iOS developer where I gathered a long list of things I didn’t know that I didn’t know. Now I’m back to doing some more self-learning with better habits.
Creating an iOS App
The first step is to open up Xcode and select “Create a new Xcode project”

Next, we select which platform our app will run on and which type of app we want to develop. We have a few different options, but in this example (the project we’ll be using going forward with this Intro to iOS Development series), the platform we’ll be running our app on is iOS and the type of app is a Single View App.

In order for us and our app to be identified when we submit our app to the App Store, we’ll need to provide some information:
- Product Name – what the name of our app will be and how it will appear in the App Store and on devices when it’s installed
- Organization Name – this organization name won’t be the same organization name that appears in the App Store, but it does appear as boilerplate text throughout our code, such as source and header file copyright text. I’m choosing my organization name to be Rising Dev Habits; your name is a viable option
- Organization Identifier – a unique identifier for the organization. When developing for ourselves, we don’t necessarily have an organization identifier. I’ll be using com.example.risingdevhabits as my identifier; this can be replaced later on when we’re ready to distribute our app. However, choose the organization identifier wisely because it is used as part of the bundle identifier, which is then used to register an App ID and the App ID can’t be changed after uploading a build to App Store Connect

Next, just before finishing creating our project, we want to make sure the Create Git repository on my Mac checkmark is unchecked. There’s nothing wrong with leaving it checked as we will be using version control in our project, but there are a couple things we can do to set up our project before putting it under version control.

This is what our project should look like after setting our configurations.

Setting Up Our Development Environment
It’s tempting to jump right into our project and start writing code. There are a few things we can do first that can save us time and headaches down the road. They are recommendations I wish I would’ve found when I first started developing for iOS and they’re also just good practice. We’ll cover the basics of getting an iOS project off the ground here. Later on I’ll put together an article with some more advanced tips to help with codebase consistency and the day-to-day processes.
Step 1: Organizing the Project
It’s easy for a project to get cluttered quickly. We can group our application delegates together, designate separate spaces for our view controllers and storyboards, and keep our assets and supporting files in their own folders as well. The idea is to not have single files living in the root of the project. Here is the basic organization system for our project; which we can and likely will make changes to as we progress and determine the architecture for our app.

Step 2: Include a README.md File
As we go along building our portfolios of iOS, watchOS, macOs, etc. projects, it’s not only helpful to document the purpose and description of the project for ourselves, but it also helps give our GitHub profile visitors an idea of what our project can do and how we did it.
Here is a post I put together with a step-by-step guide on adding a README.md to an Xcode project and what to include in it.
Step 3: Build and Run
Since we made some changes to the project’s structure when we organized it, this can sometimes cause Xcode to get confused and have trouble finding where we moved the files. So, it is good to build and run the project before putting it under version control.
Select Build in the Product tab in Xcode to build the project. Or, simply hit command + B
from the keyboard.

Troubleshooting: I noticed Xcode kept failing when attempting to build my project with a Build input file cannot be found
error due to the Info.plist file being put in the Supporting Files folder. Usually, cleaning the project, command + shift + K
, shutting down Xcode, and then reopening the project solves this issue, but this time the solution that worked for me was to pull Info.plist out of the Supporting Files folder and then build again.
To run our project, go back to the Product tab and select Run. Or, hit command + R
. When finished, you should see the simulator open up and present a blank screen.
Step 4: Add a .gitignore
The .gitignore file is used to designate which files we want tracked under source control and which ones we don’t. There will be certain files we don’t want included in our remote repository, which we will be adding in the next step. Here is a post on how to create a .gitignore file and what it could look like.
Remember when we created our project and left the Create Git repository on my Mac checkmark unchecked? Here’s why. If we were to let Xcode put our project under source control from the beginning, Git would start tracking project files (even files we don’t want tracked) and create an initial commit message. Adding a .gitignore file to our project after the fact would then require us to take measures to remove those files from the Git cache to stop tracking because files already being tracked by Git are not affected by our .gitignore file.
Step 5: Placing the Project Under Source Control
Now that we’ve set up our .gitignore file, we can place our project under source control. If you’re not already, get familiar with the basics of Git and version control.
To add Git to our project, open the terminal to the project directory and enter these git commands:
$ git init
$ git add .
$ git commit -m “Project setup”
Our project is now ready to be pushed to a remote repository.
Step 6: Create a Remote Repository and Push the Project
There are several reasons to maintain our projects in a remote repository; one of them being safety. Should something happen to our local machine, our work is saved in the remote repo. This also allows for collaboration on our projects. We can have multiple collaborators working on a project and it’ll be simple for us to push our work to and pull other’s work from the remote repository. Personally, I like using GitHub, but there are other options available such as Bitbucket or GitLab.
Here is an article on how to create a remote repository on GitHub directly through Xcode.
That’s It!
We now have an Xcode project that is setup and ready for development! Yes, this was a little more time consuming than simply creating a project and starting to code. But we got the basics out of the way that we would have had to do further down the road anyway. Getting our project setup now prevents us from having to fix or undo some work related to source control and tracking files we don’t want in our remote repository.